Today we discuss Laravel’s Browsershot integration, transforming HTML or URLs into PDF documents effortlessly. This post concentrates on generating a well-structured HTML with embedded images. Alternatively, explore creating a print service within a Docker microenvironment using browserless Chrome.
Your objective is to acquire a visually accurate PDF for storage or customer delivery. Employing relative URLs in image tags, like
<img src="/images/logo.png"/>
may cause issues with empty assets in the generated PDFs. To remedy this, utilize an absolute link path instead:
<img src="https://johndoe.com/images/logo.png" />
.
Today we discuss Laravel’s Browsershot integration, transforming HTML or URLs into PDF documents effortlessly. This post concentrates on generating a well-structured HTML with embedded images. Alternatively, explore creating a print service within a Docker microenvironment using browserless Chrome.
Your objective is to acquire a visually accurate PDF for storage or customer delivery. Employing relative URLs in image tags, like
<img src="/images/logo.png"/>
may cause issues with empty assets in the generated PDFs. To remedy this, utilize an absolute link path instead:
<img src="https://johndoe.com/images/logo.png" />
.
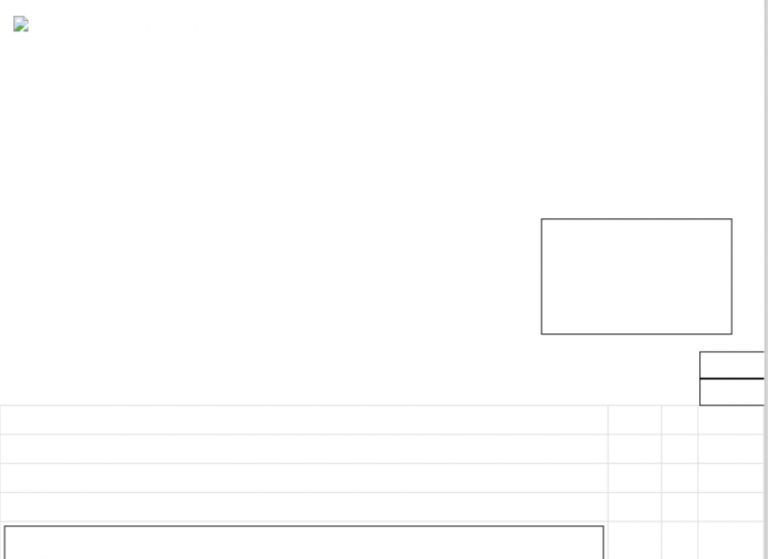
In Laravel you can use a URL helper if you are not sure what the URL is going to be or you want your code to be extensible on any site.
<img src="{{ URL::asset('/images/logo.png') }}">
For context, one might employ this technique when the need arises to create a PDF snapshot of a webpage for storage purposes or client distribution. To avoid empty assets in generated PDFs, it’s crucial to utilize absolute URL paths instead of relative ones for image sources. In Laravel, an elegant way is to leverage URL helpers, ensuring compatibility regardless of the application’s domain.
Let’s illustrate this with a comprehensive example. Suppose one creates an invoice in a Blade template:
...
<div class="col-xs-3">
<div>
<p></p>
<img src="{{ URL::asset('/images/logo.png') }}" height="35" alt="Business Logo" />
</div>
</div>
<table class="table table-striped table-bordered table-condensed">
<thead>
<tr>
<th>Description</th>
<th>Price</th>
<th>Qty</th>
<th>Amount</th>
</tr>
</thead>
<tbody>
@foreach($products as $product)
<tr>
<td>{{ $product->name }}</td>
<td>${{ $product->price }}</td>
<td>{{ $product['qty'] }}</td>
<td>${{ $product['qty'] * $product['price'] }}</td>
</tr>
@endforeach
</tbody>
</table>
...
In the controller, one would call this template and subsequently process it with Browsershot to obtain a PDF:
// use Spatie\Browsershot\Browsershot;
public function getInvoicePdf(Request $request, $id) {
$invoice = Invoice::findOrFail($id);
$products = $invoice->products;
$html = view("sales.invoice", compact("products"));
$pdf = Browsershot::html($html) ->windowSize(1920, 1080) // Optional - set desired dimensions of the rendered page
->waitUntilNetworkIdle(true) // Ensure all assets have loaded before capturing the PDF
->pdf();
$headers = array('Content-Type: application/pdf');
return Response::download($pdf, "invoice-{$invoice->id}.pdf", $headers);
}
Feel free to provide feedback if you come across a specific use case requiring further elaboration. By optimizing code readability and context, the revised blog aims to clarify the usage of Laravel Browsershot in generating PDFs with images intact.